For Loop
for(/*initialization*/ ;/*condition*/ ;/*afterthought*/)
{
//action that will run in loop
}
//example
for(var i=0; i < 10; i+=1)
{
console.log("Hello world");
}
//this code above will run 10 times, i=0, i=1, , , i=9
It tells the computer what to do, and how to do it.
- Initialization – it happens at the very beginning of the for loop
- Condition – determines when the for loop ends
- Afterthought – happens after the loop ends
function setup()
{
createCanvas(800,800);
for(var i = 0; i < 100; i += 1)
{
//console.log("Hello World!");
fill(random(0, 255));
ellipse(random(100, 800), random(100, 800), 100, 100);
}
}
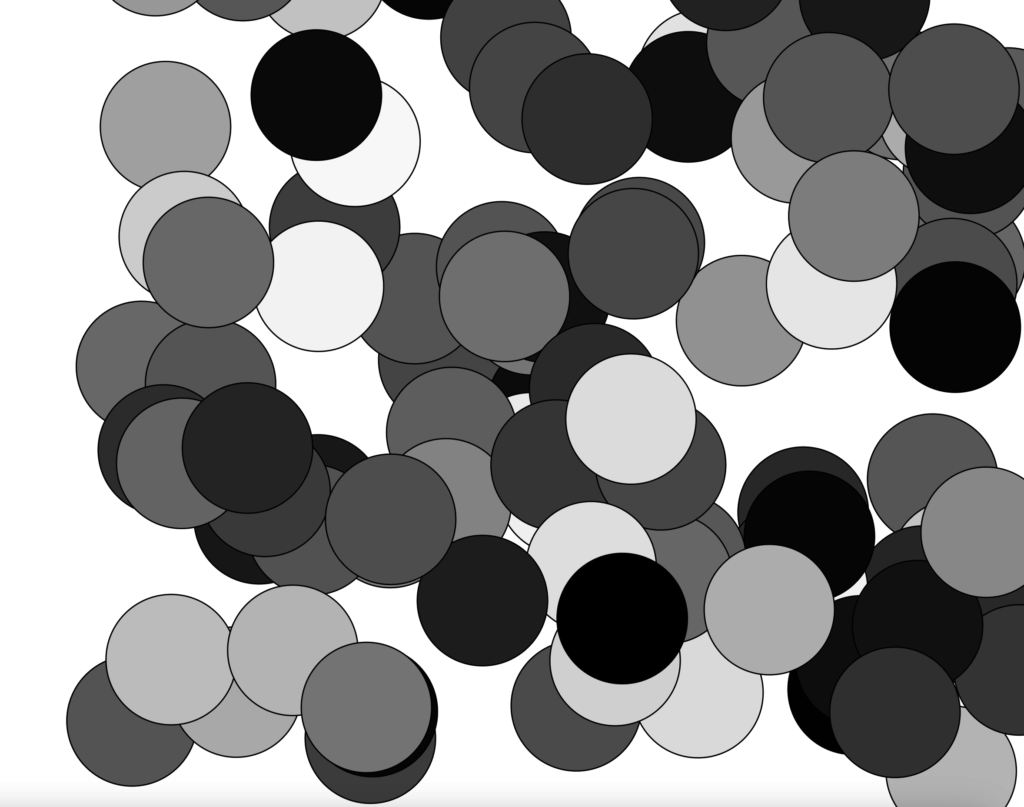
function draw()
{
background(255);
for(var i = 0; i < mouseX; i += 1)
{
//console.log("Hello World!");
fill(random(0, 255));
ellipse(random(0, 800), random(0, 800), 100, 100);
}
}
Draw does a self-loop, so this continuously does the loop.
//From Exercises
var sum = 0;
function setup()
{
createCanvas(800,800);
for(var i = 0; i < 10; i += 1)
{
//console.log("Hello World!");
// fill(random(0, 255));
// ellipse(random(100, 800), random(100, 800), 100, 100);
// rect(random(100), random(100), 10, 10);
// ellipse(random(width), 100, 50, 50);
// line(0, 0, random(width), random(height));
sum = sum + 1;
}
for(var i = 100; i > 0; i -= 1)
{
sum = sum - 1;
}
console.log(sum);
}
//function draw()
//{
//// background(255);
//// for(var i = 0; i < mouseX; i += 1)
//// {
//// //console.log("Hello World!");
//// fill(random(0, 255));
//// ellipse(random(0, 800), random(0, 800), 100, 100);
//// }
////}
Example from the lesson today, that I wanted to review more later:
var x_pos;
function setup()
{
createCanvas(800,800);
}
function draw()
{
background(0);
x_pos = random(1,100);
fill(255, 0, 255);
for(var i=0; i < 20; i ++ /*same as i +=1*/)
{
ellipse(x_pos, 100, 20, 20);
x_pos += 30;
console.log(x_pos);
}
}
var x_pos;
var y_pos
function setup()
{
createCanvas(800,800);
}
function draw()
{
background(0);
x_pos = 100;
y_pos = 100;
fill(255, 0, 255);
for(var i=0; i < 20; i ++ /*same as i +=1*/)
{
ellipse(x_pos, y_pos, 20, 20);
x_pos += 30;
y_pos += 30;
console.log(x_pos);
}
}
Variable i or index variable
var x_pos;
var y_pos
function setup()
{
createCanvas(800,800);
}
function draw()
{
background(0);
x_pos = 100;
y_pos = 100;
fill(255, 0, 255);
for(var i=0; i < 20; i ++ /*same as i +=1*/)
{
ellipse(100 + i * 30, 100 + i * 30, 20, 20); //no brackets needed, MDAS was followed
// x_pos += 30;
// y_pos += 30;
// console.log(x_pos);
}
}
10×10 grid square
for(var i = 0; i < 11; i ++)
{
line(i * 10, 0, i * 10, 100);
line(0, i * 10, 100, i * 10);
}
Combining for loops with conditional statements
function setup()
{
createCanvas(800,600);
strokeWeight(2);
}
function draw()
{
background(235);
noFill();
stroke(150);
for(var i = 0; i < 9; i++)
{
var r = 0;
if(i > 7)
{
stroke(220, 200, 255);
}
else if(i > 3)
{
stroke(255, 100, 0);
r = 20;
}
else if(i > 1)
{
stroke(155, 0, 100);
r = 5;
}
else
{
stroke(150);
}
rect(80+i*80 + random(-r, r), 80 + random(-r, r), 60, 60); // the width of the square is 60px, which gives an exact 20px distance in between squares
console.log(80+i*80); //the x point is every 80*(1, 2, 3, 4)
}
for(var i = 0; i < 9; i++)
{
var r = 0;
if(i > 6)
{
stroke(220, 200, 255);
}
else if(i > 4)
{
stroke(255, 100, 0);
r = 20;
}
else if(i > 0)
{
stroke(155, 0, 100);
r = 5;
}
else
{
stroke(150);
}
rect(80+i*80 + random(-r, r), 160 + random(-r, r), 60, 60); // the width of the square is 60px, which gives an exact 20px distance in between squares
console.log(80+i*80); //the x point is every 80*(1, 2, 3, 4)
}
}
Exercises to Explain
var x = 0;
var y = 0;
var len = 100;
for(var i = 0; i < len; i++)
{
if(i < len / 2)
{
x++;
}
else
{
y++;
}
}
console.log("(" + x + ", " + y + ")")
for(var i = 1; i <= 300; i++)
{
if(i < 100)
{
stroke(255, 0, 0);
}
else if(i < 200)
{
stroke(0, 255, 0);
}
else
{
stroke(0, 0, 255);
}
point(i, 0);
console.log(i);
}
for(var i = 1; i < 1000; i++) //999 iterations
{
if(i < 200) // 1-199 = 199
{
ellipse(random(width), random(height), 20, 20);
}
else // 999 iterations - 199 (i < 200) = 800
{
rect(random(width), random(height), 20, 20);
}
if(i >= 800) // 800 to 999 = 199
{
ellipse(random(width), random(height), 20, 20);
}
// point(i, 0);
}