Array
- a type of variable
- a list of items (booleans, strings, numbers)
- a data structure
Object
var button =
{
X: 200,
Y: 200,
Width: 100,
Height: 50,
}
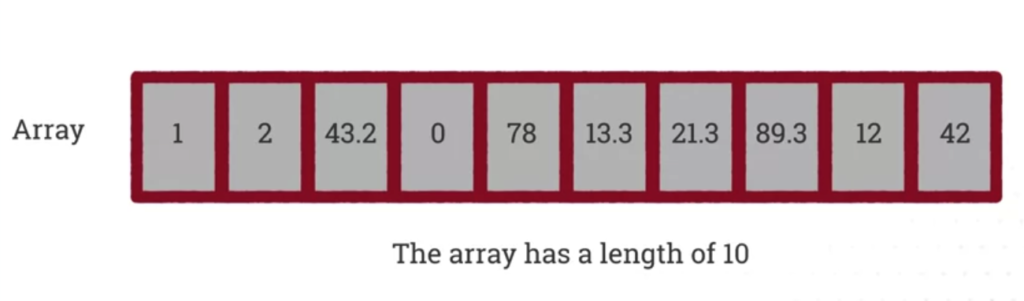
Each element of the array is indexed.
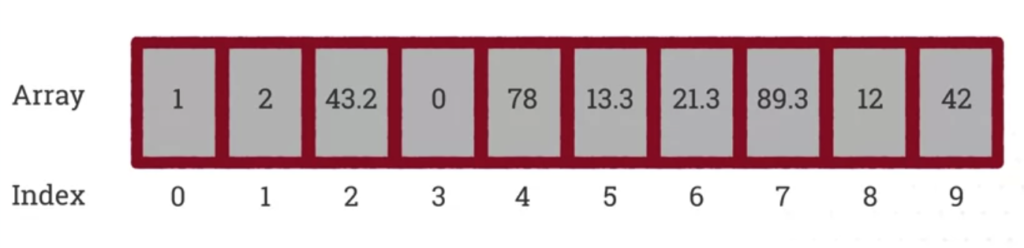
Object vs Array
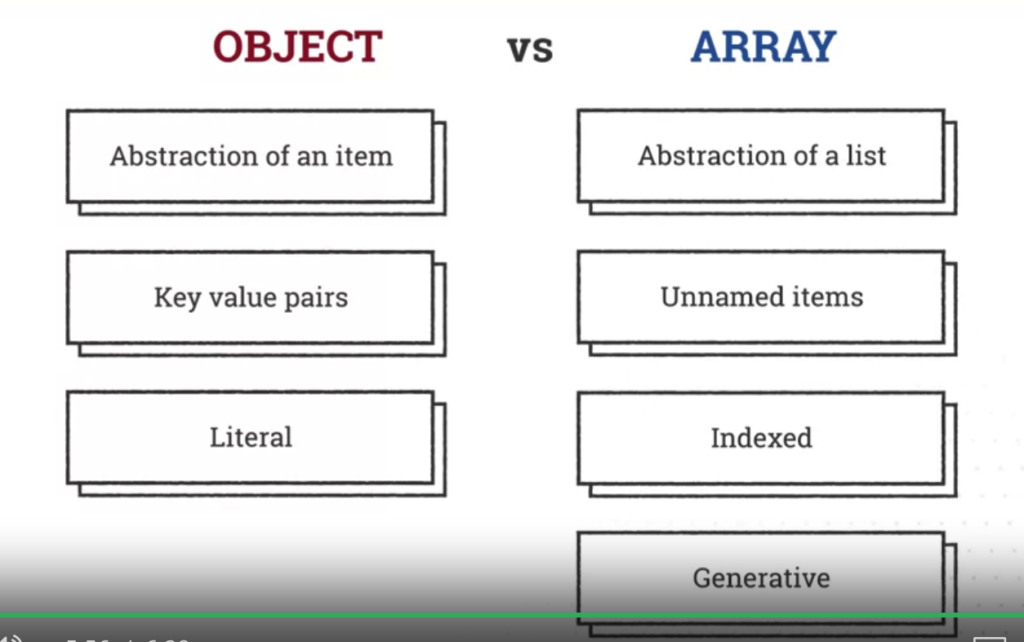
When to use an array
- list of related values
- normally of the same type
Coding with Arrays
var myArrayNumbers = [1,2,3,4,5,6,7,8]
var myArrayStrings = ["hello", "world", "goodbye", "earth"]
var myArrayMixed = [1,2.32, "hello", false]
var myArrayEmpty = [];
To call an element in an array
var myArray = [1/*0*/, 2/*1*/, 43.2/*2*/, 0/*3*/, 78/*4*/, 13.3/*5*/, 21.3/*6*/, 89.3/*7*/, 12/*8*/, 42/*9*/];
console.log(myArray[0]); //1
console.log(myArray[5]); //13.3
myArray[5] == 100; //replaced array 5 from 13.3 to 100
console.log(myArray[5]); //100
console.log(myArray[10]); //undefined, our list is only till variable 9
Example from the lesson
var sizes = [4, 9, 10, 5, 110, 95, 40, 40];
var names = ["Mercury", "Venus", "Earth", "Mars", "Jupiter", "Saturn", "Uranus", "Neptune"];
var colours = ["gray", "brown", "red", "sienna", "pink", "blue", "cyan"];
function setup()
{
createCanvas(1000, 600);
}
function draw()
{
background(0);
textAlign(CENTER);
// fill(255);
fill(colours[0]);
ellipse(50, height/2, sizes[0]); //used "sizes" because that's the name of the array
fill(255);//color of text
text(names[0], 50, height/2 + 150);//first element in the array for names, same x as the planet, and the height is 150px lower
fill(colours[1]);
ellipse(175, height/2, sizes[1]);
fill(255);//color of text
text(names[1], 175, height/2 + 150);//first element in the array for names, same x as the planet, and the height is 150px lower
fill(colours[2]);
ellipse(300, height/2, sizes[2]);
fill(255);//color of text
text(names[2], 300, height/2 + 150);//first element in the array for names, same x as the planet, and the height is 150px lower
fill(colours[3]);
ellipse(425, height/2, sizes[3]);
fill(255);//color of text
text(names[3], 425, height/2 + 150);//first element in the array for names, same x as the planet, and the height is 150px lower
fill(colours[4]);
ellipse(550, height/2, sizes[4]);
fill(255);//color of text
text(names[4], 550, height/2 + 150);//first element in the array for names, same x as the planet, and the height is 150px lower
fill(colours[5]);
ellipse(675, height/2, sizes[5]);
fill(255);//color of text
text(names[5], 675, height/2 + 150);//first element in the array for names, same x as the planet, and the height is 150px lower
fill(colours[6]);
ellipse(800, height/2, sizes[6]);
fill(255);//color of text
text(names[6], 800, height/2 + 150);//first element in the array for names, same x as the planet, and the height is 150px lower
fill(colours[7]);
ellipse(925, height/2, sizes[7]);
fill(255);//color of text
text(names[7], 925, height/2 + 150);//first element in the array for names, same x as the planet, and the height is 150px lower
}
//IMPORTANT NOTE
//To list colors in RGB
var colours = [[128, 128, 128], [165, 42, 42], [255, 0, 0], [136, 45, 23], [255, 192, 203], [0, 0, 255], [0, 255, 255]];
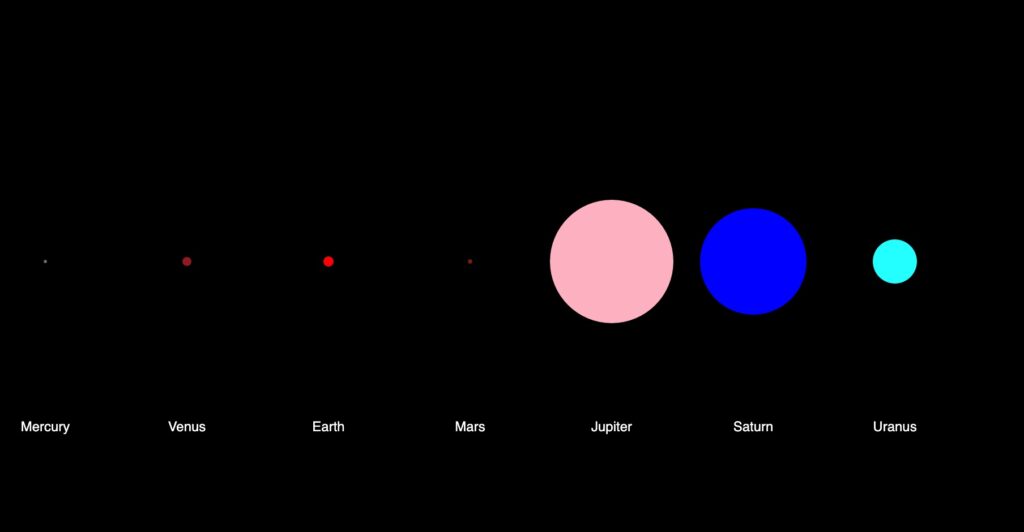
What happened here?
var a = [2, 0, 1];
var b = [20, 30, 40]
if( b[a[0]] == 40) // this means (b[2] == 40), (b[40] == 40)
{
console.log("Access Granted!");
}
//to resolve it
var a = [2, 0, 1];
var b = [20, 30, 40]
if( b[a[0]] == 40)
{
console.log("Access Granted!");
}
else
{
console.log("value of the b array is the [a[0]], which is 40");
}
Common Bugs
- Array should use [] and not {}
- no empty array in the end, don’t put extra .
- Don’t enter infinite loop
As a practice, I worked on the planets code at the top and simplify it.
var sizes = [4, 9, 10, 5, 110, 95, 40, 40];
var names = ["Mercury", "Venus", "Earth", "Mars", "Jupiter", "Saturn", "Uranus", "Neptune"];
var colours = [[128, 128, 128], [165, 42, 42], [255, 0, 0], [136, 45, 23], [255, 192, 203], [0, 0, 255], [0, 255, 255]];
var ellipse_x = [50, 175, 300, 425, 550, 675, 800, 925]; //added this variable to represent the x position of the ellipse
function setup()
{
createCanvas(1000, 600);
}
function draw()
{
background(0);
textAlign(CENTER);
for(var i = 0; i < 8; i++)
{
fill(colours[i]);
ellipse(ellipse_x[i], height/2, sizes[i]);
fill(255);
text(names[i], ellipse_x[i], height/2 + 150);
// console.log(i);
}
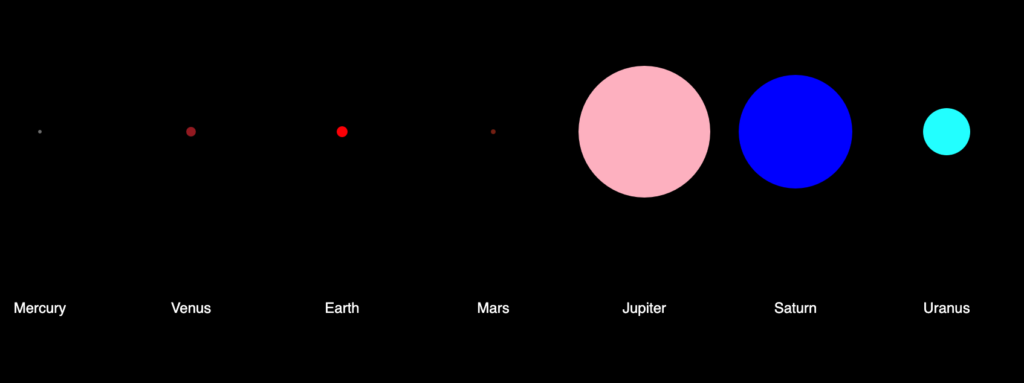