- HTML – structures website content
- CSS – applies styling to websites
- JavaScript – adds interactivity to websites
- SQL – allows your web application to store and retrieve data
HTML
- the skeleton of all web pages
- provides structure to the content
- HyperText Markup Language
Markup
Markup refers to a way of annotating text that is distinguishable from the text itself.
HTML separates content and annotation by using HTML tags, which are denoted by angle brackets (also known as less-than and greater-than signs).
HTML Elements
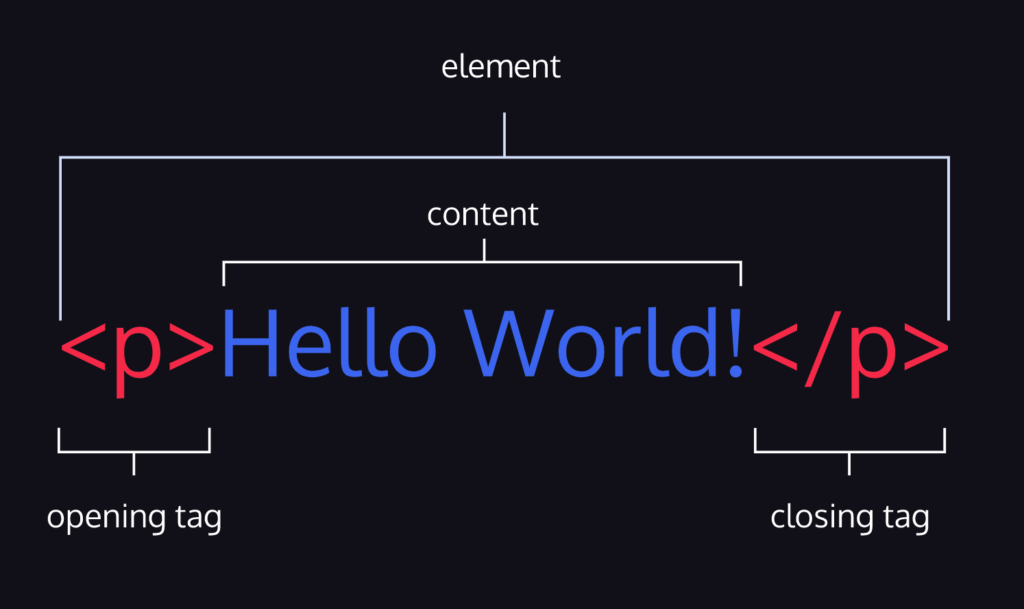
- HTML element — a unit of content in an HTML document formed by HTML tags and the text or media it contains.
- Opening Tag — the element name used to start an HTML element. The tag type is surrounded by opening and closing angle brackets.
- Content — The information (text or other elements) contained between the opening and closing tags of an HTML element.
- Closing tag — the second HTML tag used to end an HTML element. Closing tags have a forward slash (/) inside of them, directly after the left angle bracket.
<h1>This is a heading, it emphasizes text.</h1>
<p>This is a paragraph, it is the most common tag for larger chunks of text.</p>
<a>This is an anchor tag, used to specify the text that is the "anchor" for a link.</a>
<button>This is a button.</button>
HyperText
- Text that is linked to other text.
- hyper indicates that the text expands beyond the traditional constraints of the written word.
Adding Hyperlinks
An attribute in HTML provides additional information about an HTML element.
name="value"
Example:
width="500"
href
attribute – hyperlink reference. Specify the URL, or address on the web, that a link should take users to.
<a href="https://www.codecademy.com">Learn to code!</a>
relative path – a link to another page on the same website, so we can omit the first part. Instead of www.jetsetter.com/resume.html
we can abbreviate the link to just /resume.html
.
<a href="/resume.html">25 years of experience</a>
CSS
- language that provides style to the content of an HTML page.
Computer Science principle separation of concerns
selectors specify the HTML elements to which the style should be applied
visual rules specify how that content should be displayed.
HTML link tag – used to create a connection between an HTML file and the CSS file and tells the browser to apply the CSS styles to the HTML.
<link rel="stylesheet" href="style.css">
JavaScript
Add website features:
- popup ads
- animated graphics (2D or 3D)
- interactive audio and video
- maps and other features access the user’s geographic location
- and much more!
User interaction:
- the user clicking on a button
- the user pressing enter on their keyboard
- a video file finishing loading
- the user re-sizing their window
- the user hovering over text on the webpage
JavaScript Functions
- Functions allow us to write a chunk of code once that can be reused over and over.
- Events allow JavaScript to respond to user behaviors, like the user hovering their mouse over an HTML element or resizing their window.
<script>
function getRandomColor(){
let letters = '0123456789ABCDEF';
let color = '#';
for (let i = 0; i < 6; i++) {
color += letters[Math.floor(Math.random() * 16)];
}
return color;
}
function changeColor(){
let newColor = getRandomColor();
document.body.style.backgroundColor = newColor;
}
</script>
First, the getRandomColor()
function generates a random series of numbers and letters to create a new hexadecimal color code.
It then saves the new color under the name newColor
.
let newColor = getRandomColor();
On the next line, the document
keyword accesses the page’s background color and sets it equal to the new color.
document.body.style.backgroundColor = newColor;
HTML attributes can set events, where the name of the attribute is the event and the value of the attribute is the JavaScript function that we want to execute.
<body>
<button onclick="changeColor()">Repaint!</button>
</body>
<script>
function getRandomColor(){
let letters = '0123456789ABCDEF';
let color = '#';
for (let i = 0; i < 6; i++) {
color += letters[Math.floor(Math.random() * 16)];
}
return color;
}
function changeColor(){
let newColor = getRandomColor();
document.body.style.backgroundColor = newColor;
}
</script>
SQL
SQL stands for structured query language. It stores information in tables, which is simply a collection of information organized into rows and columns.
query is a program that would retrieve data from the table.
SELECT * FROM page_views;
JavaScript on the Web
JavaScript, also called JS, is a flexible and powerful language that is implemented consistently by various web browsers, making it the language for web development. JavaScript, HTML, and CSS are the core components of web technology. While HTML is responsible for structure and CSS is responsible for style, JavaScript provides interactivity to web pages in the browser.
- JavaScript can be used in both the front-end and back-end of web development.
- JavaScript is standardized so it’s frequently updated with new versions.
- JavaScript integrates easily with HTML and CSS.
- JavaScript allows websites to have interactivity like scroll transitions and object movement. Modern browsers still compete to process JavaScript the fastest for the best user experiences. Chrome, the most used Internet browser in 2017, has been so successful because of its ability to process JavaScript quickly.
- JavaScript offers a wide range of frameworks and libraries that help developers create complex applications with low overhead. Programmers can import libraries and frameworks in their code to augment their application’s functionality.
Node.JS, or Node, is one of the most popular versions of server-side JavaScript. Node has been used to write large platforms for NASA, eBay and many others. Since Javascript can execute programs out of sequential order, Node can be used to create scalable web applications, messaging platforms, and multiplayer games. This is why Google Cloud and Amazon Web Service depend on Node for some of their services.
We use some popular standalone desktop apps like Slack, GitHub, Skype, and Tidal. These applications are developed with the JavaScript framework called Electron.js.
Leave a Reply