Use i < 199
, rather <= 199
to start at the last index (198) to 0.
//array length 199
var metamorphosis = ["One", "morning,", "when", "Gregor", "Samsa", "woke", "from", "troubled", "dreams,", "he", "found", "himself", "transformed", "in", "his", "bed", "into", "a", "horrible", "vermin.", "He", "lay", "on", "his", "armour-like", "back,", "and", "if", "he", "lifted", "his", "head", "a", "little", "he", "could", "see", "his", "brown", "belly,", "slightly", "domed", "and", "divided", "by", "arches", "into", "stiff", "sections.", "The", "bedding", "was", "hardly", "able", "to", "cover", "it", "and", "seemed", "ready", "to", "slide", "off", "any", "moment.", "His", "many", "legs,", "pitifully", "thin", "compared", "with", "the", "size", "of", "the", "rest", "of", "him,", "waved", "about", "helplessly", "as", "he", "looked.", "'What's", "happened", "to", "me?'", "he", "thought.", "It", "wasn't", "a", "dream.", "His", "room,", "a", "proper", "human", "room", "although", "a", "little", "too", "small,", "lay", "peacefully", "between", "its", "four", "familiar", "walls.", "A", "collection", "of", "textile", "samples", "lay", "spread", "out", "on", "the", "table", "-", "Samsa", "was", "a", "travelling", "salesman", "-", "and", "above", "it", "there", "hung", "a", "picture", "that", "he", "had", "recently", "cut", "out", "of", "an", "illustrated", "magazine", "and", "housed", "in", "a", "nice,", "gilded", "frame.", "It", "showed", "a", "lady", "fitted", "out", "with", "a", "fur", "hat", "and", "fur", "boa", "who", "sat", "upright,", "raising", "a", "heavy", "fur", "muff", "that", "covered", "the", "whole", "of", "her", "lower", "arm", "towards", "the", "viewer.", "Gregor", "then", "turned", "to", "look", "out", "the", "window", "at", "the", "dull", "weather."];
function setup()
{
// for(var i = 0;i < 199; i++)
// {
// console.log(metamorphosis[i]);
// }
createCanvas(1000, 900);
background(50);
}
function draw()
{
fill(255);
textSize(40);
var cursorX = 0;
var cursorY = 40;
for(var i = 0;i < 199; i++)
{
text(metamorphosis[i], cursorX, cursorY);
cursorX += textWidth(metamorphosis[i] + " ");
if(cursorX > (width - 100))
{
cursorY += 40;
cursorX = 0;
}
}
}
Example – planets from the previous lesson:
//var sizes = [4, 9, 10, 5, 110, 95, 40, 40];
//var names = ["Mercury", "Venus", "Earth", "Mars", "Jupiter", "Saturn", "Uranus", "Neptune"];
//var colours = [[128, 128, 128], [165, 42, 42], [255, 0, 0], [136, 45, 23], [255, 192, 203], [0, 0, 255], [0, 255, 255]];
var planets =
[
{size: 4, name: "Mercury", colour: "gray"},
{size: 9, name: "Venus", colour: "brown"},
{size: 10, name: "Earth", colour: "blue"},
{size: 5, name: "Mars", colour: "red"},
{size: 110, name: "Jupiter", colour: "sienna"},
{size: 95, name: "Saturn", colour: "pink"},
{size: 40, name: "Uranus", colour: "blue"},
{size: 40, name: "Neptune", colour: "cyan"},
]
function setup()
{
createCanvas(1000, 600);
}
function draw()
{
background(0);
textAlign(CENTER);
fill(255);
for(var i = 0; i < 8; i++)
{
fill(planets[i].colour);
ellipse(50 + i*125, height/2, planets[i].size);//increase the value of x from 50 for the first planet, and increase it 125 each time
fill(255);
text(planets[i].name, 50 + i*125, height/2 + 150);
}
}
Arrays can be compared with each other.
var fib = [0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144]
fill(255);
for(var i = 1; i < 12; i++)
{
if(fib[i-1] + fib[i] == fib[i+1])
{
line(fib[i], 0, fib[i], height);
console.log(fib);
}
}
var gaps = [1, 3, 9, 12];
for(var i = 0; i < 100; i++)
{
var x = gaps[round(random(0, 4))];
line(x, 0, x, height);
console.log([i]);
}
Nested Iteration for drawing patterns
Nested iteration to draw grids
function setup()
{
createCanvas(1200, 600);
}
function draw()
{
background(0);
fill(255, 0, 255);
for(var h = 0; h < mouseY/10; h++)//rows the 10 value that was divided from mouseY determines the size of the area
{
for(var i = 0; i < mouseX/10; i++)
{
ellipse(30 + i * 30,30 + h * 30, 30, 30); //the value of 3o that was multipled from the i and h determines the spaces in between the ellipses
}
}
}
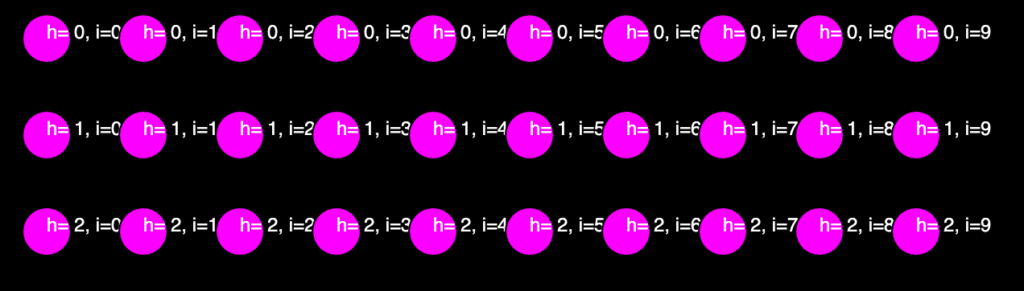
function draw()
{
background(0);
fill(255, 0, 255);
for(var h = 0; h < 3; h++)//rows
{
for(var i = 0; i < 10; i++)
{
fill(255, 0, 255);
ellipse(30 + i * 60,30 + h * 60, 30, 30); //columns
fill(255);
text("h= " + h + ", i=" + i, /*the values of h and i*/
30 + i * 60, /*text's x position*/
30 + h * 60) /*text's y position*/
}
}
}
Console Debugger to check how the for loop
is working
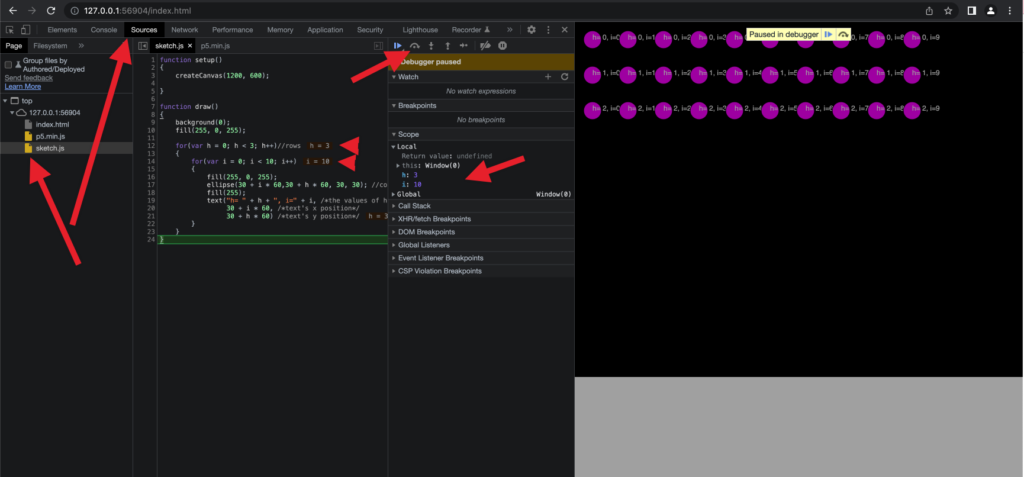
Exercises
for(var i = 0; i < 10; i++)// 10 * 1 = 10 - width
{
for(var j = 0; j < 10; j++) //10 * 1 = 10 height
{
strokeWeight(3);
fill(255, 0, 255);
point(random(width), random(height)); // number of points 10 * 10
}
}
for(var i = 0; i < 100; i += 10) // grid width 100/10 = 10
{
for(var j = 0; j < 10; j += 2) // grid height 10/2 = 5
{
point(i, j); // 10 * 5 = 50
}
}
//returns 50 posint drawn in a grid that is 90 pixels wide and 9 pixels high, why?