This would be the third course I’d be taking for CSS Flexbox. I really liked the one from Scrimba so far, compared with the one from Udacity.com. I think Per did a really great job on structuring the course, and delivering it (This is speaking from a trainer ad instructor’s perspective.)
This third course I am taking for Flexbox is from Treehouse. I wanted to see different perspective on this, and have some hands-on later one.
By the way today is 3 days after Christmas, and 3 days before New Year! 😊 I am so thankful, I am on my holiday leaves.
Flexbox – flexible box layout. It is a set of CSS properties that proved a flexible way to layout page content.
- Flex Container
- sets the context for flexbox layout
- contains flex items, the actual elements you layout using flexbox
- can be any block-level or inline element
- Flex Item
- every direct child of a flex container
- there can be any number of flex items inside a flex container
Axes
Flexbox follows two axis that determine the layout direction of Flex items. Everything in a flexbox layout is relative to these two axes.
- Main axis – primary axis along which Flex items are laid out. It defines the direction of the Flex items in the Flex container. The default is left to right.
- Cross axis – runs perpendicular to the main axis. The default direction is top to bottom.
By default, display flex creates a block level flex container.
display: inline-flex;
– the container follows the width of the items
display: flex;
– the container has a default width, which doesn’t changed regardless of the width of the items inside.
Any elements outside the flex container is not affected.
Controlling the Direction of Flex Items
By default, flex items are laid out horizontally on the main axis from left to right.
flex-direction: row;
default direction of the flex items, from left to right.
flex-direction: row-reverse;
reverse the direction from right to left, and is right aligned.
flex-direction: column;
changes the direction from horizontal to vertical, changes the main axis
flex-direction: column-reverse;
reverses the direction in column
Flex Wrap Property
By default, the flex container lays out flex items on a single line called flex line. The flex container tries to fit all items on one flex line even if it causes its contents to overflow.
The flex wrap property lets you control whether the flex container is a single line or multi-line layout.
flex-wrap: wrap;
/* wrap, no-wrap, wrap-reverse */
Justify Property
justify-content property lets you control the position and alignment of flex items on the main axis, and how space will be distributed in a flex container.
Default is justify-content: flex-start;

justify-content: flex-start;


justify-content: center;


Order Property
By default, the flex items are displayed in order how they were coded on the HTML file.
The order
property will let you manage the ordering of the flex items without changing the code in the HTML file.
The default order of every flex item is 0
. In order to place one item to the first, it should have an order less than 0
or -1
.
order: 2;
must be added to the individual flex item on CSS file.
.container {
display: flex;
flex-wrap: wrap;
justify-content: space-around;
}
.item-1 {
margin-right: auto;
}
.item-6 {
order: -1;
}
.item-5 {
order: 1;
}
.item-2 {
order: 2;
}

Flex Grow Property
By default, the flex items don’t fill the entire flex line. If there are less flex items, they will just be aligned to the left by default.
flex-grow:
will let you fill up the entire flex line.
The default value for flex-grow
is 0, or it will not expand.
.container > .item {
flex-grow: 1;
}
/* this is the same with */
.item {
flex-grow: 1;
}
/* item class was added to individual flex items, but I think I would use the first one */

This can be useful when working with content area and sidebar. Set flex-grow for each, so to make them responsive. flex-grow
values are from 1, 2, 3, and so on.
Flex Basis Property
It works alongside the flex-grow
property to display equal, ut flexible width for all items.
It accepts the same values as the width
and height
-properties – pixels, ems, percentages, and other units.
flex-basis: 200px;
The flex items will display at an equal size, when they’re 200pixels or wider, but if the screen gets narrower the browser will redistribute the space.
Flex Shorthand Property
flex
– is the shorthand of flex-grow,
flex-shrink
, and flex-basis
.
.container {
display: flex;
flex-wrap: wrap;
}
.item-1 {
flex: 1 150px;
}
.item-2 {
flex: 3 500px;
}
/*This is how the sidebar goes down to the bottom for the narrower screen like mobile phones. */
Cross Axis
The cross axis runs perpendicular to the main axis.
- The
align-items
property applies to flex containers only. - The
align-self
property applies to flex items only. align-items
aligns flex items vertically in the flex container.- To align all flex items to the start of the cross axis, use the
align-items: flex-start;
. align-items: flex-end;
packs the items toward the end of the cross axis.align-items: center;
perfectly centers items along the cross axis.align-self: flex-start;
aligns a flex item to the start of the cross axis.align-self: flex-end;
aligns a flex item to the end of the cross axis.align-self: center;
aligns a flex item to the center of the cross axis.
Three ways to center-align elements using flexbox:
Set the flex container’s justify-content
and align-items
values to center
:
.container {
justify-content: center;
align-items: center;
}
Set the flex container’s justify-content
value to center
, while setting the flex item’s align-self
value to center
:
.container {
justify-content: center;
}
.item {
align-self: center;
}
Set the flex item’s margin
value to auto
:
.item {
margin: auto;
}
Building a Navigation Bar with Flexbox
Media Queries
/* =================================
Media Queries
==================================== */
/*Mobile phones*/
@media (min-width: 769px) {
}
/* Desktop */
@media (min-width: 1025px) {
}
https://d.pr/i/I87CHL/c7MUOoUq1g
/* =================================
Media Queries
==================================== */
@media (min-width: 769px) {
.main-header, .main-nav {
display: flex;
}
.main-header {
flex-direction: column; /* this was added, because there are three hierarchy on this flex container - .main-header > .main-nav, and name > and the contents inside, therefore from here, I am not changing the individual menu items, but only the title (name) and the menu set (.main-nav) */
align-items: center;
}
}
@media (min-width: 1025px) {
.main-header { /* this was added, because there are three hierarchy on this flex container - .main-header > .main-nav, and name > and the contents inside, therefore from here, I am not changing the individual menu items, but only the title (name) and the menu set (.main-nav) */
flex-direction: row;
justify-content: space-between;
}
}
Aligning Items to the Bottom of a Column
- It’s possible for an element to be both a flex item and a flex container.
- A
margin
value ofauto
has an effect on flex item alignment because it absorbs any extra space around a flex item and pushes other flex items into different positions.
Working with elements inside columns
/* =================================
Media Queries
==================================== */
@media (min-width: 769px) {
.main-header,
.main-nav,
.row,
.col { /* this is a flex container */
display: flex;
}
.main-header {
flex-direction: column;
align-items: center;
}
.col { /*this is a flex container and flex item at the same time */
flex: 1;
flex-direction: column;
}
.button { /*this is the flex item of the .col container */
margin-top: auto;
align-self: center;
}
@media (min-width: 1025px) {
.main-header {
flex-direction: row;
justify-content: space-between;
}
}
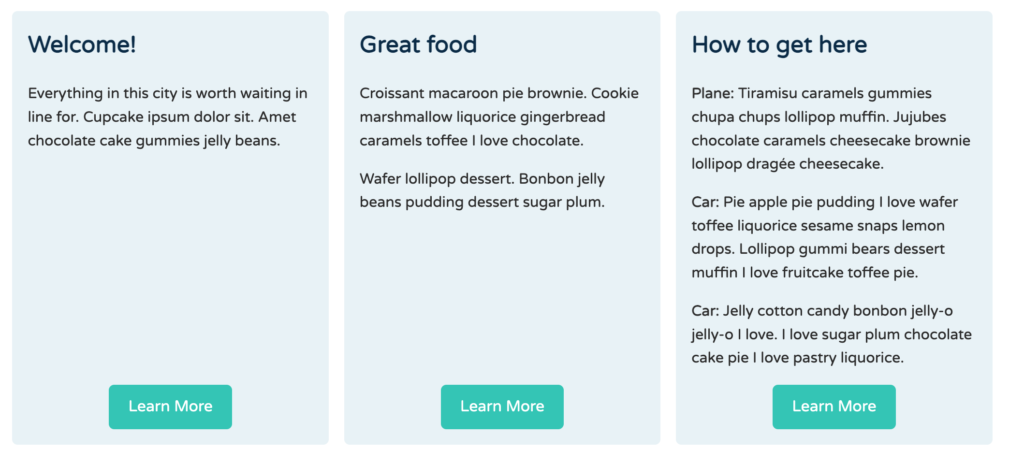
Resources
- A Complete Guide to Flexbox
- Flexbox – latest browser support
- Flexbox Froggy – A fun way to practice flexbox
- Flexbugs – A community-curated list of flexbox issues and cross-browser workarounds for them
I like Guil among all instructors I watched so far on this. Guil presented it professionally, though Per is good in explaining complex things in a simpler and more understandable manner.
Leave a Reply