Variable
- one of the most important concepts in programming
- a way of storing and keeping track of information in a program, so that it can be later used, and manipulated.
- score is an example of a variable
var score;
/* Declaring a variable */
var score = 0;
/* Assigning a value to a variable */
Declaring a Variable
var message = "Hello!";
console.log(message);
In this program, the word “message” is now a reference to the value “Hello!”, so whenever I use “message” in the program, as long as I don’t change its value, it will always mean, “Hello!”.
Reassigning the Variable
var message = "Hello!";
console.log(message);
message = "Hello from JavaScript Basics";
We don’t use var
again to reassign a variable, it’s only used when we first create or declare a variable.
Naming Variables
- JavaScript has unique words that you can’t use for a variable name, so if you encounter a syntax error when creating a variable, this might be the reason.
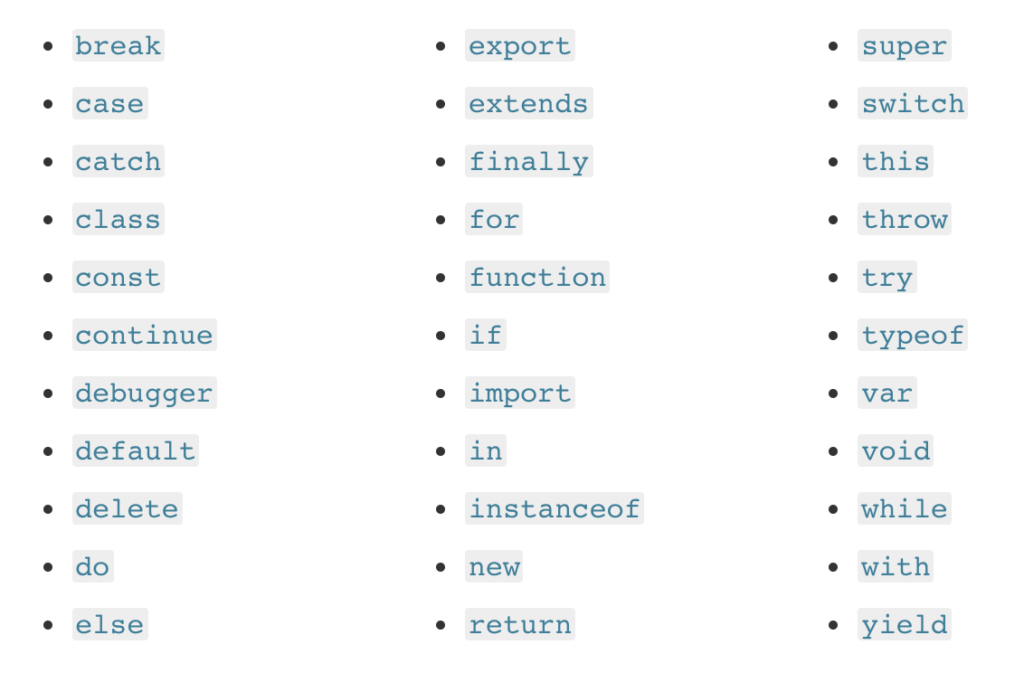
- Names can’t start with a number.
- Names can only contain letters, numbers, and the
$
and_
characters. - Capitalizing every word following the first is called camel case by programmers
Changing the Value in a Variable
var score = 0
score = score + 10;
- When putting a value into a variable, whatever’s to the right of the equal sign goes into the variable on the left.
- In this case above, what’s the right is the current contents of the variable score + 10.
var score = 0
score += 10; /* addition assignment operator - addition to its current value */
/* this adds a value 10 to the current value of the score */
var bonusPts = 100;
var finalScore = score + bonusPts;
console.log(finalScore);
/* We are adding the current value of the variable score plus the value of the variable bonusPts and saving the sum of those values to the third variable, finalScore */
let
and const
const
– constant, the value of the variable can’t change. We cannot change nor manipulate the value of a constant through reassignment. We also cannot redeclare a constant.
let
– for variables whose value should change while the program runs. let
won’t let you override an assignment, while var
would let you reassign a variable
Practice
// 1. Attach this file -- practice.js -- to the index.html file using a <script> tag
<script src="practice.js"></script>
// 2. In this JavaScript file, add a prompt dialog to capture input from the user and store it in a variable
const firstName = prompt('What is your first name?');
// 3. Add a second a prompt dialog to capture input from the user and store it in a second variable
const lastName = prompt('What is your last name?');
// 4. Create a third variable and which combines an uppercase version values in the two other variables separated by a space. For example, if the first two variables contain "sally" and "forth", this third variable should contain the string value "SALLY FORTH"
const fullName = firstName + " " + lastName;
const fullNameUppercase = fullName.toUpperCase();
alert(fullNameUppercase);
// 5. Create a fourth variable to store a number. The number should be the total number of characters in the third variable.
let charCount = fullName.trim().length;
// 6. Add an alert dialog box that says "The string '[insert value of third variable here]' is X number of characters long." For example, if the third variable contained the string "SALLY FORTH" then the alert dialog should says "The string 'SALLY FORTH' is 11 characters long."
alert("The string \"" + fullNameUppercase + "\" is " + charCount + " characters long.");
Resources:
- Addition (
+
) – MDN - [Addition assignment operator – MDN](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Addition_assignment
let
– MDNconst
– MDN