fr
– fraction unit, a flexible length unit designed for creating grid tracks that expand and contract based on the free space in the grid.
auto
– can expand a column to fill up extra space. It sizes items based on content, so it doesn’t give you total control over how much space an item takes up.
CSS Grid can intelligently control the fluidity or responsiveness of your layout. This means you can set items to automatically wrap and adjust to fit their container’s width. It makes it possible to create flexible grid layouts without media queries.
1fr
represents one fraction of the available space in the grid container. The free space that an fr
takes up is calculated after any non flexible tracks.
.grid {
max-width: 1000px;
display: grid;
grid-template-columns: 200px auto 200px;
grid-template-rows: 100px 200px;
grid-gap: 15px;
}
/* USING FR */
.grid {
max-width: 1000px;
display: grid;
grid-template-columns: 1fr 1fr 1fr; /* fr */
grid-template-rows: 100px 200px;
grid-gap: 15px;
}
repeat() Notation
The CSS Grid repeat()
function saves you time and helps keep your CSS maintainable. repeat()
offers a shortcut for repeating patterns of tracks, which keeps you from having to write the same values over and over again.
.grid {
max-width: 1000px;
display: grid;
grid-template-columns: repeat(3, 1fr); /*this repeats 1fr 3 times*/
grid-template-rows: 100px 200px;
grid-gap: 15px;
}
/*another example*/
.grid {
max-width: 1000px;
display: grid;
grid-template-columns: 2fr repeat(3, 1fr); /*this gives first column 2fr, and then repeats 1fr 3 times for the succeeding columns*/
grid-template-rows: 100px 200px;
grid-gap: 15px;
}
Flexible Track Sizing with minmax()
minmax()
lets you set a grid track’s minimum and maximum size. For example, you might give certain tracks a minimum size to make sure the content doesn’t become too narrow or short and set a max size to ensure tracks don’t get too wide or tall, or expand to fit the content that might be added later. And it makes it possible to create responsive Grid Layout without the ease of media queries.
minmax()
the first value is the minimum, and the second value is the maximum
.grid {
max-width: 1000px;
display: grid;
grid-template-columns: minmax(200px, 1fr) 1fr 1fr; /*The minimum width of the first column is 200px, and the maximum is 300px */
grid-template-rows: 100px 200px;
grid-gap: 15px;
}
*Note the minmax()
here is applied per column.
.grid {
max-width: 1000px;
display: grid;
grid-template-columns: minmax(200px, 1fr) minmax(200px, 1fr) minmax(200px, 1fr); /*The minimum width of each 3 columns is 200px, and the maximum is 300px */
grid-template-rows: 100px 200px;
grid-gap: 15px;
}
/*this can be simplified with repeat notation as follow: */
.grid {
max-width: 1000px;
display: grid;
grid-template-columns: repeat(3, minmax(200px, 1fr));
grid-template-rows: 100px 200px;
grid-gap: 15px;
}
Repeat Tracks with auto-fill and auto-fit
Grid layout lets you set items to automatically wrap and adjust to fit their container’s width. This lets you create designs that respond to different browser widths. The Grid Layout repeat
notation supports two keywords — auto-fill
and auto-fit
— to help with this.
.grid {
max-width: 1000px;
display: grid;
grid-template-columns: repeat(auto-fill, 200px); /*instead of forcing the browser to display a specific number of columns, this will auto-fill with number of columns with 200px max width*/
grid-template-rows: 100px 200px;
grid-gap: 15px;
}
To remove extra spaces on the right side, we can combine minmax
with auto-fill to distribute any remaining space among the 60px columns.
.grid {
max-width: 1000px;
display: grid;
grid-template-columns: repeat(auto-fill, minmax(60px, 1fr)); /*this distributes any remaining space to each columns for wider screens, however this may still cause extra space if lesser items*/
grid-template-rows: 100px 200px;
grid-gap: 15px;
}
*auto-fill
generates as many columns as necessary to fit the items within the grid container width even if it means generating empty tracks.
*auto-fit
collapses any empty tracks down to zero pixels. Grid items can expand to take up the remaining space.
.grid {
max-width: 1000px;
display: grid;
grid-template-columns: repeat(auto-fit, minmax(60px, 1fr)); /*this collapses any empty tracks to 0, so the not empty tracks will collapse to fit the container size*/
grid-template-rows: 100px 200px;
grid-gap: 15px;
}
The Explicit and Implicit Grid
When you create a grid layout with the grid-template-rows
and grid-template-columns
properties, you are defining what’s called an “explicit grid”. The implicit grid is automatically generated by the grid container to position any extra items outside of the explicit grid.
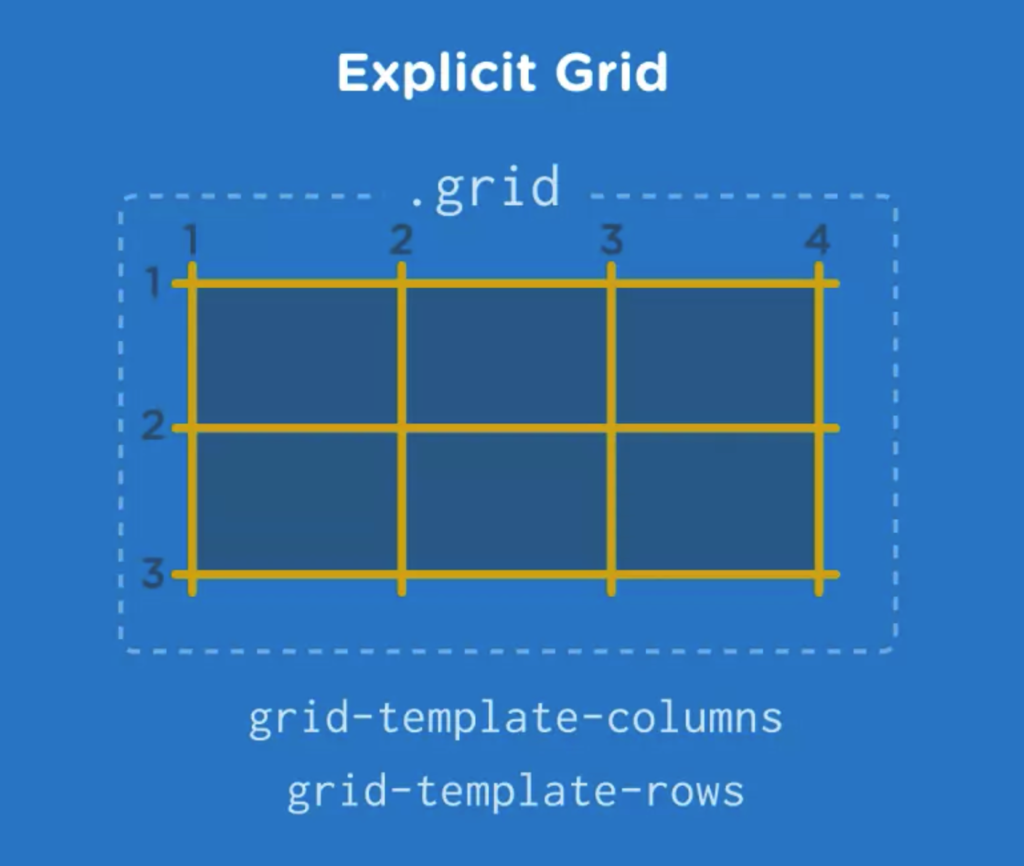
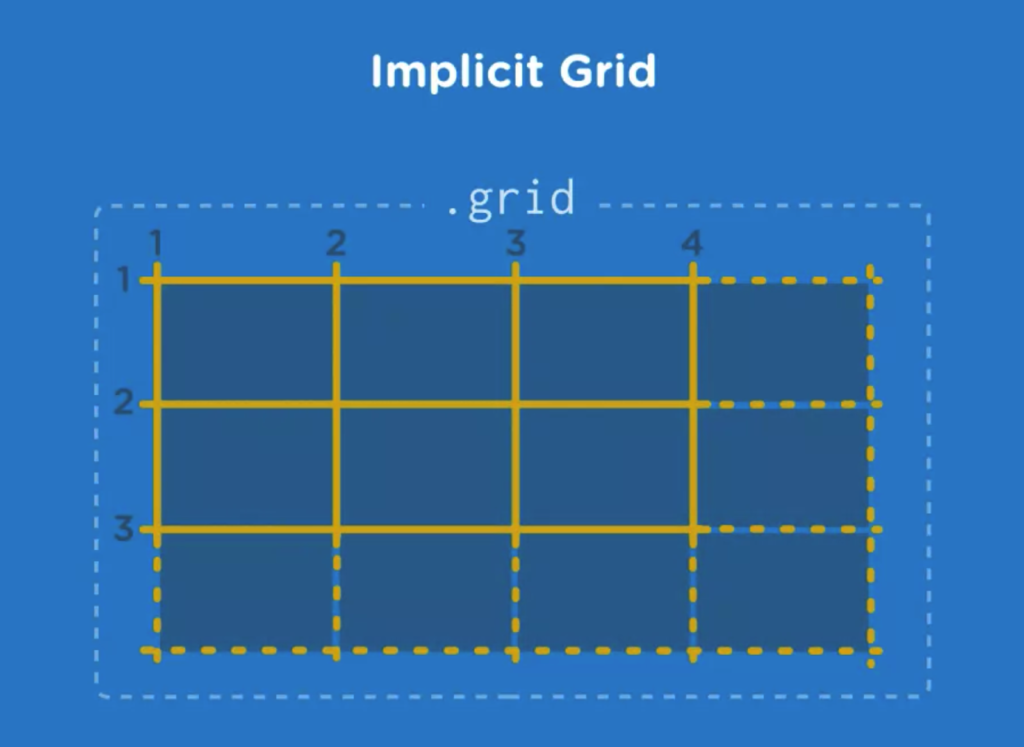
Implicit grids are resized automatically by content.
Control the Auto-placement of Grid Items
Grid layout generates implicit grid tracks to position any items outside of the explicit grid.
Control Implicit Tracks
grid-auto-rows controls
implicit row tracksgrid-auto-columns controls
implicit column tracks
.grid {
max-width: 1000px;
display: grid;
grid-template-columns: 1fr 1fr 1fr;
grid-template-rows: 100px 200px; /*explicit grid*/
grid-gap: 15px;
grid-auto-rows: 250px; /*implicit grid */
}
In this example above, the first row takes 100px height, second row is 200px height (explicit grid), then all the other rows (implicit grid) takes 250px height.
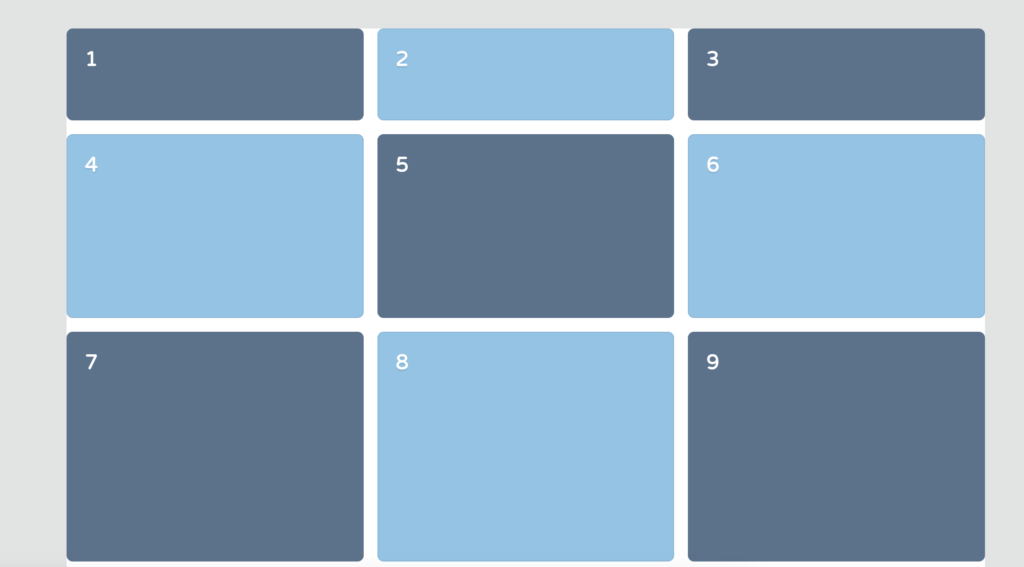
.grid {
max-width: 1000px;
display: grid;
grid-template-columns: 1fr 1fr 1fr;
/*grid-template-rows: 100px 200px;*/ /*this removes the explicit rows*/
grid-gap: 15px;
grid-auto-rows: 250px;
}
In this example above, the explicit grid were deleted, hence all the rows now follow the implicit grid size of 250px.
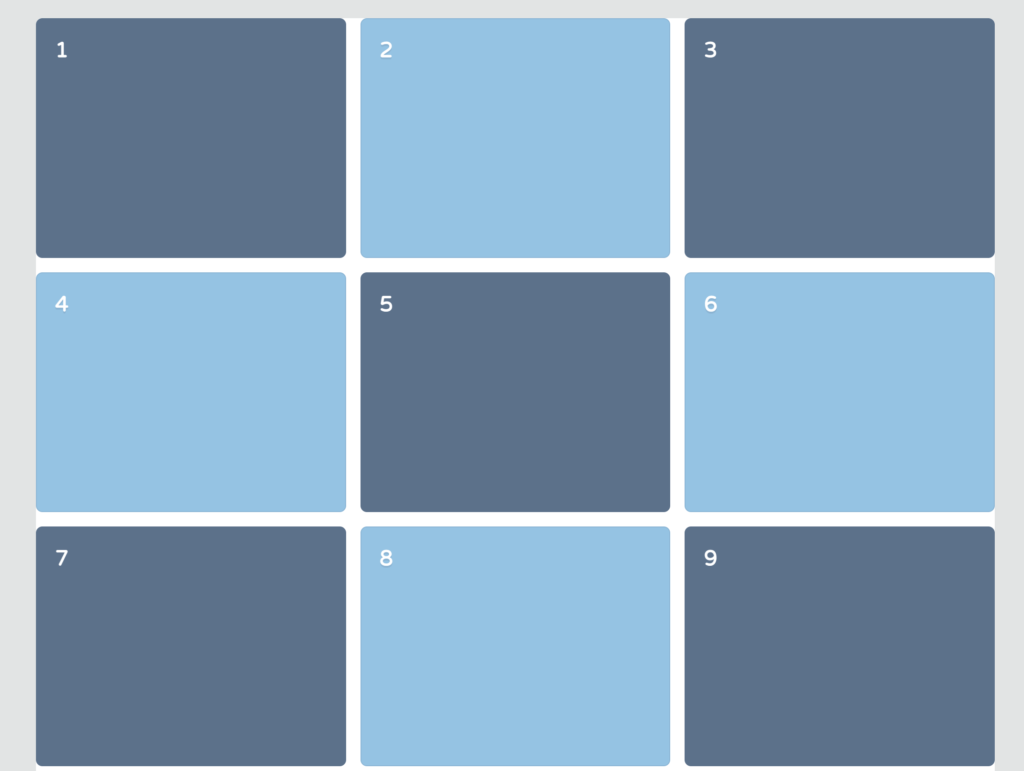
To create implicit rows that are a minimum size but then grow to fit more content, we can use minmax
function.
.grid {
max-width: 1000px;
display: grid;
grid-template-columns: 1fr 1fr 1fr;
grid-template-rows: 100px 100px;
grid-gap: 15px;
grid-auto-columns: 1fr;
grid-auto-flow: column;
}
/* =====================================================================
Practice CSS Grid Layout
======================================================================== */
.main-content {
width: 95%;
max-width: 1000px;
display: grid;
grid-template-columns: repeat(auto-fit, minmax(280px, 1fr));
grid-gap: 20px;
}
Resources:
- Track listings with
repeat()
notation – MDN repeat()
– MDN- Track sizing and
minmax()
– MDN minmax()
– MDNrepeat()
values – MDN- The implicit and explicit grid – MDN
- The Difference Between Explicit and Implicit Grids
grid-auto-rows
– MDNgrid-auto-columns
– MDN- The implicit and explicit grid – MDN
grid-auto-rows
– MDNgrid-auto-columns
– MDN- The implicit and explicit grid – MDN
- Grid Layout – MDN
- CSS Grid Layout – Treehouse course
Leave a Reply